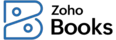
IN THIS PAGE…
Get Supported Entity
Organization
To fetch the current organization’s details:
ZFAPPS.get('organization').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
organization: {
organization_id: "ORG_ID",
org_name: "ORG_NAME",
org_mode: "TEST",
org_email: "ORG_EMAIL"
}
}
ZFAPPS.get('organization.organization_id').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"organization.organization_id": "ORG_ID"
}
Supported Keys:
Property | Request |
---|---|
organization_id | organization.organization_id |
org_email | organization.org_email |
org_mode | organization.org_mode |
org_name | organization.org_name |
data_center_extension | organization.data_center_extension |
User
To fetch the details of the user accessing the widget:
ZFAPPS.get('user').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
user: {
user_id: "USER_ID",
name: "USER_NAME",
email: "USER_EMAIL",
zuId: "USER_ZUID"
}
}
ZFAPPS.get('user.name').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"user.name": "USER_NAME"
}
Property | Request |
---|---|
user_id | user.user_id |
name | user.name |
user.email | |
zuId | user.zuId |
Invoice
Invoice details
This method lets you fetch the details of an invoice. This can be used only when the widget is displayed in the invoice details or creation page.
To fetch the details of an invoice:
ZFAPPS.get('invoice').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"invoice": {
"invoice_id": "112233",
"invoice_number": "INV-000006",
"salesorder_id": "",
"salesorder_number": "",
"crm_owner_id": "",
"zcrm_potential_id": "",
"zcrm_potential_name": "",
"date": "2018-08-25",
"date_formatted": "25 Aug 2019",
"status": "paid",
"status_formatted": "Paid",
"amount": 101,
"amount_formatted": "$101.00",
.......
}
}
This method lets you fetch the list of invoices. This can be used only when the widget is displayed in the invoices list page.
ZFAPPS.get('invoices').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"invoices": [
{
"invoice_id": "112233",
"invoice_number": "INV-000006",
...
},
{
"invoice_id": "112233",
"invoice_number": "INV-000006",
...
}
....
]
}
Supported Keys:
Property | Request |
---|---|
invoice_id | invoice.invoice_id |
invoice_number | invoice.invoice_number |
salesorder_id | invoice.salesorder_id |
salesorder_number | invoice.salesorder_number |
crm_owner_id | invoice.crm_owner_id |
zcrm_potential_id | invoice.zcrm_potential_id |
zcrm_potential_name | invoice.zcrm_potential_name |
date | invoice.date |
date_formatted | invoice.date_formatted |
status | invoice.status |
status_formatted | invoice.status_formatted |
autobill_status | invoice.autobill_status |
autobill_status_formatted | invoice.autobill_status_formatted |
payment_terms | invoice.payment_terms |
payment_terms_label | invoice.payment_terms_label |
due_date | invoice.due_date |
due_date_formatted | invoice.due_date_formatted |
payment_expected_date | invoice.payment_expected_date |
payment_expected_date_formatted | invoice.payment_expected_date_formatted |
payment_discount | invoice.payment_discount |
payment_discount_formatted | invoice.payment_discount_formatted |
last_payment_date | invoice.last_payment_date |
last_payment_date_formatted | invoice.last_payment_date_formatted |
reference_number | invoice.reference_number |
customer_id | invoice.customer_id |
estimate_id | invoice.estimate_id |
contact_category | invoice.contact_category |
customer_name | invoice.customer_name |
unused_credits_receivable_amount | invoice.unused_credits_receivable_amount |
unused_credits_receivable_amount_formatted | invoice.unused_credits_receivable_amount_formatted |
unused_retainer_payments | invoice.unused_retainer_payments |
unused_retainer_payments_formatted | invoice.unused_retainer_payments_formatted |
contact_persons | invoice.contact_persons |
currency_id | invoice.currency_id |
currency_code | invoice.currency_code |
currency_symbol | invoice.currency_symbol |
exchange_rate | invoice.exchange_rate |
discount | invoice.discount |
discount_applied_on_amount | invoice.discount_applied_on_amount |
discount_type | invoice.discount_type |
recurring_invoice_id | invoice.recurring_invoice_id |
documents | invoice.documents |
line_items | invoice.line_items |
submitter_id | invoice.submitter_id |
approver_id | invoice.approver_id |
submitted_date | invoice.submitted_date |
submitted_date_formatted | invoice.submitted_date_formatted |
submitted_by | invoice.submitted_by |
contact_persons_details | invoice.contact_persons_details |
salesorders | invoice.salesorders |
deliverychallans | invoice.deliverychallans |
shipping_charge | invoice.shipping_charge |
shipping_charge_formatted | invoice.shipping_charge_formatted |
adjustment | invoice.adjustment |
adjustment_formatted | invoice.adjustment_formatted |
roundoff_value | invoice.roundoff_value |
roundoff_value_formatted | invoice.roundoff_value_formatted |
adjustment_description | invoice.adjustment_description |
transaction_rounding_type | invoice.transaction_rounding_type |
sub_total | invoice.sub_total |
sub_total_formatted | invoice.sub_total_formatted |
tax_total | invoice.tax_total |
tax_total_formatted | invoice.tax_total_formatted |
discount_total | invoice.discount_total |
discount_total_formatted | invoice.discount_total_formatted |
total | invoice.total |
total_formatted | invoice.total_formatted |
discount_percent | invoice.discount_percent |
bcy_shipping_charge | invoice.bcy_shipping_charge |
bcy_adjustment | invoice.bcy_adjustment |
bcy_sub_total | invoice.bcy_sub_total |
bcy_discount_total | invoice.bcy_discount_total |
bcy_tax_total | invoice.bcy_tax_total |
bcy_total | invoice.bcy_total |
taxes | invoice.taxes |
payment_made | invoice.payment_made |
payment_made_formatted | invoice.payment_made_formatted |
credits_applied | invoice.credits_applied |
credits_applied_formatted | invoice.credits_applied_formatted |
balance | invoice.balance |
balance_formatted | invoice.balance_formatted |
write_off_amount | invoice.write_off_amount |
write_off_amount_formatted | invoice.write_off_amount_formatted |
payment_options | invoice.payment_options |
contact_persons_associated | invoice.contact_persons_associated |
payments | invoice.payments |
shipping_bills | invoice.shipping_bills |
credits | invoice.credits |
comments | invoice.comments |
billing_address | invoice.billing_address |
shipping_address | invoice.shipping_address |
customer_default_billing_address | invoice.customer_default_billing_address |
notes | invoice.notes |
terms | invoice.terms |
custom_fields | invoice.custom_fields |
template_id | invoice.template_id |
template_name | invoice.template_name |
template_type | invoice.template_type |
template_type_formatted | invoice.template_type_formatted |
created_time | invoice.created_time |
last_modified_time | invoice.last_modified_time |
created_date | invoice.created_date |
created_date_formatted | invoice.created_date_formatted |
created_by_id | invoice.created_by_id |
last_modified_by_id | invoice.last_modified_by_id |
salesperson_id | invoice.salesperson_id |
salesperson_name | invoice.salesperson_name |
merchant_id | invoice.merchant_id |
merchant_name | invoice.merchant_name |
ecomm_operator_id | invoice.ecomm_operator_id |
ecomm_operator_name | invoice.ecomm_operator_name |
invoice_url | invoice.invoice_url |
bills | invoice.bills |
sub_total_inclusive_of_tax | invoice.sub_total_inclusive_of_tax |
sub_total_inclusive_of_tax_formatted | invoice.sub_total_inclusive_of_tax_formatted |
subject_content | invoice.subject_content |
approvers_list | invoice.approvers_list |
zoho_recruit_jobopening_id | invoice.zoho_recruit_jobopening_id |
Estimate
Estimate details
This method lets you fetch the details of an estimate. This can be used only when the widget is displayed in the estimate details or creation page.
To fetch the details of an estimate:
ZFAPPS.get('estimate').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"estimate": {
"estimate_id": "112233",
"estimate_number": "INV-000006",
"salesorder_id": "",
"salesorder_number": "",
"crm_owner_id": "",
"zcrm_potential_id": "",
"zcrm_potential_name": "",
"date": "2018-08-25",
"date_formatted": "25 Aug 2019",
"status": "paid",
"status_formatted": "Paid",
"amount": 101,
"amount_formatted": "$101.00",
.......
}
}
Note: Refer the API Docs to know the list key available for estimate detail
Estimate list
This method lets you fetch the list of estimates. This can be used only when the widget is displayed in the estimates list page.
ZFAPPS.get('estimates').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"estimates": [
{
"estimate_id": "112233",
"estimate_number": "INV-000006",
...
},
{
"estimate_id": "112233",
"estimate_number": "INV-000006",
...
}
....
]
}
Note: Refer the API Docs to know the list key available for estimate list
Supported Keys:
Property | Request |
---|---|
estimate_id | estimate.estimate_id |
estimate_number | estimate.estimate_number |
crm_owner_id | estimate.crm_owner_id |
crm_custom_reference_id | estimate.crm_custom_reference_id |
zcrm_potential_id | estimate.zcrm_potential_id |
zcrm_potential_name | estimate.zcrm_potential_name |
date | estimate.date |
date_formatted | estimate.date_formatted |
created_date | estimate.created_date |
created_date_formatted | estimate.created_date_formatted |
reference_number | estimate.reference_number |
status | estimate.status |
status_formatted | estimate.status_formatted |
customer_id | estimate.customer_id |
documents | estimate.documents |
customer_name | estimate.customer_name |
place_of_supply | estimate.place_of_supply |
place_of_supply_formatted | estimate.place_of_supply_formatted |
vat_treatment | estimate.vat_treatment |
contact_category | estimate.contact_category |
tax_treatment | estimate.tax_treatment |
tax_treatment_formatted | estimate.tax_treatment_formatted |
contact_persons | estimate.contact_persons |
currency_id | estimate.currency_id |
currency_code | estimate.currency_code |
currency_symbol | estimate.currency_symbol |
exchange_rate | estimate.exchange_rate |
expiry_date | estimate.expiry_date |
expiry_date_formatted | estimate.expiry_date_formatted |
discount | estimate.discount |
discount_applied_on_amount | estimate.discount_applied_on_amount |
is_discount_before_tax | estimate.is_discount_before_tax |
discount_type | estimate.discount_type |
is_viewed_by_client | estimate.is_viewed_by_client |
client_viewed_time | estimate.client_viewed_time |
client_viewed_time_formatted | estimate.client_viewed_time_formatted |
is_inclusive_tax | estimate.is_inclusive_tax |
tax_rounding | estimate.tax_rounding |
estimate_url | estimate.estimate_url |
line_items | estimate.line_items |
submitter_id | estimate.submitter_id |
submitted_date | estimate.submitted_date |
submitted_date_formatted | estimate.submitted_date_formatted |
submitted_by | estimate.submitted_by |
approver_id | estimate.approver_id |
shipping_charge | estimate.shipping_charge |
shipping_charge_formatted | estimate.shipping_charge_formatted |
bcy_shipping_charge | estimate.bcy_shipping_charge |
adjustment | estimate.adjustment |
adjustment_formatted | estimate.adjustment_formatted |
bcy_adjustment | estimate.bcy_adjustment |
adjustment_description | estimate.adjustment_description |
roundoff_value | estimate.roundoff_value |
roundoff_value_formatted | estimate.roundoff_value_formatted |
transaction_rounding_type | estimate.transaction_rounding_type |
sub_total | estimate.sub_total |
sub_total_formatted | estimate.sub_total_formatted |
bcy_sub_total | estimate.bcy_sub_total |
sub_total_inclusive_of_tax | estimate.sub_total_inclusive_of_tax |
sub_total_inclusive_of_tax_formatted | estimate.sub_total_inclusive_of_tax_formatted |
sub_total_exclusive_of_discount | estimate.sub_total_exclusive_of_discount |
sub_total_exclusive_of_discount_formatted | estimate.sub_total_exclusive_of_discount_formatted |
discount_total | estimate.discount_total |
discount_total_formatted | estimate.discount_total_formatted |
bcy_discount_total | estimate.bcy_discount_total |
discount_percent | estimate.discount_percent |
total | estimate.total |
total_formatted | estimate.total_formatted |
bcy_total | estimate.bcy_total |
tax_total | estimate.tax_total |
tax_total_formatted | estimate.tax_total_formatted |
bcy_tax_total | estimate.bcy_tax_total |
price_precision | estimate.price_precision |
taxes | estimate.taxes |
comments | estimate.comments |
invoice_ids | estimate.invoice_ids |
billing_address | estimate.billing_address |
shipping_address | estimate.shipping_address |
customer_default_billing_address | estimate.customer_default_billing_address |
notes | estimate.notes |
terms | estimate.terms |
custom_fields | estimate.custom_fields |
template_id | estimate.template_id |
template_name | estimate.template_name |
template_type | estimate.template_type |
template_type_formatted | estimate.template_type_formatted |
created_time | estimate.created_time |
last_modified_time | estimate.last_modified_time |
created_by_id | estimate.created_by_id |
last_modified_by_id | estimate.last_modified_by_id |
contact_persons_associated | estimate.contact_persons_associated |
contact_persons_details | estimate.contact_persons_details |
salesperson_id | estimate.salesperson_id |
salesperson_name | estimate.salesperson_name |
attachment_name | estimate.attachment_name |
allow_partial_payments | estimate.allow_partial_payments |
payment_options | estimate.payment_options |
payments | estimate.payments |
subject_content | estimate.subject_content |
approvers_list | estimate.approvers_list |
Contact
Contact details
This method lets you fetch the details of an contacts. This can be used only when the widget is displayed in the contact details or creation page.
To fetch the details of an contact:
ZFAPPS.get('contact').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"contact": {
"contact_id": "112233",
"contact_number": "987776575",
.......
}
}
Contact list
This method lets you fetch the list of contacts. This can be used only when the widget is displayed in the contacts list page.
ZFAPPS.get('contacts').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"contacts": [
{
"contact_id": "112233",
"contact_number": "987776575",
...
},
{
"contact_id": "112233",
"contact_number": "887776572",
...
}
....
]
}
Supported Keys:
Property | Request |
---|---|
contact_id | contact.contact_id |
contact_name | contact.contact_name |
company_name | contact.company_name |
first_name | contact.first_name |
last_name | contact.last_name |
designation | contact.designation |
department | contact.department |
website | contact.website |
language_code | contact.language_code |
language_code_formatted | contact.language_code_formatted |
contact_salutation | contact.contact_salutation |
contact.email | |
phone | contact.phone |
mobile | contact.mobile |
portal_status | contact.portal_status |
has_transaction | contact.has_transaction |
contact_type | contact.contact_type |
customer_sub_type | contact.customer_sub_type |
customer_sub_type_formatted | contact.customer_sub_type_formatted |
owner_id | contact.owner_id |
owner_name | contact.owner_name |
source | contact.source |
source_formatted | contact.source_formatted |
documents | contact.documents |
contact.twitter | |
contact.facebook | |
is_crm_customer | contact.is_crm_customer |
is_linked_with_zohocrm | contact.is_linked_with_zohocrm |
is_gapps_customer | contact.is_gapps_customer |
primary_contact_id | contact.primary_contact_id |
zcrm_account_id | contact.zcrm_account_id |
zcrm_contact_id | contact.zcrm_contact_id |
crm_owner_id | contact.crm_owner_id |
payment_terms | contact.payment_terms |
payment_terms_label | contact.payment_terms_label |
credit_limit_exceeded_amount | contact.credit_limit_exceeded_amount |
credit_limit_exceeded_amount_formatted | contact.credit_limit_exceeded_amount_formatted |
currency_id | contact.currency_id |
currency_code | contact.currency_code |
currency_symbol | contact.currency_symbol |
price_precision | contact.price_precision |
exchange_rate | contact.exchange_rate |
opening_balance_amount | contact.opening_balance_amount |
opening_balance_amount_formatted | contact.opening_balance_amount_formatted |
opening_balance_amount_bcy | contact.opening_balance_amount_bcy |
opening_balance_amount_bcy_formatted | contact.opening_balance_amount_bcy_formatted |
outstanding_receivable_amount | contact.outstanding_receivable_amount |
outstanding_receivable_amount_formatted | contact.outstanding_receivable_amount_formatted |
outstanding_receivable_amount_bcy | contact.outstanding_receivable_amount_bcy |
outstanding_receivable_amount_bcy_formatted | contact.outstanding_receivable_amount_bcy_formatted |
outstanding_payable_amount | contact.outstanding_payable_amount |
outstanding_payable_amount_formatted | contact.outstanding_payable_amount_formatted |
outstanding_payable_amount_bcy | contact.outstanding_payable_amount_bcy |
outstanding_payable_amount_bcy_formatted | contact.outstanding_payable_amount_bcy_formatted |
unused_credits_receivable_amount | contact.unused_credits_receivable_amount |
unused_credits_receivable_amount_formatted | contact.unused_credits_receivable_amount_formatted |
unused_credits_receivable_amount_bcy | contact.unused_credits_receivable_amount_bcy |
unused_credits_receivable_amount_bcy_formatted | contact.unused_credits_receivable_amount_bcy_formatted |
unused_credits_payable_amount | contact.unused_credits_payable_amount |
unused_credits_payable_amount_formatted | contact.unused_credits_payable_amount_formatted |
unused_credits_payable_amount_bcy | contact.unused_credits_payable_amount_bcy |
unused_credits_payable_amount_bcy_formatted | contact.unused_credits_payable_amount_bcy_formatted |
unused_retainer_payments | contact.unused_retainer_payments |
unused_retainer_payments_formatted | contact.unused_retainer_payments_formatted |
status | contact.status |
payment_reminder_enabled | contact.payment_reminder_enabled |
is_sms_enabled | contact.is_sms_enabled |
is_portal_enabled | contact.is_portal_enabled |
is_client_review_settings_enabled | contact.is_client_review_settings_enabled |
custom_fields | contact.custom_fields |
is_taxable | contact.is_taxable |
tax_id | contact.tax_id |
tax_name | contact.tax_name |
tax_percentage | contact.tax_percentage |
country_code | contact.country_code |
country_code_formatted | contact.country_code_formatted |
contact_category | contact.contact_category |
contact_category_formatted | contact.contact_category_formatted |
is_linked_with_contact | contact.is_linked_with_contact |
sales_channel | contact.sales_channel |
ach_supported | contact.ach_supported |
portal_receipt_count | contact.portal_receipt_count |
opening_balances | contact.opening_balances |
billing_address | contact.billing_address |
shipping_address | contact.shipping_address |
contact_persons | contact.contact_persons |
comments | contact.comments |
addresses | contact.addresses |
pricebook_id | contact.pricebook_id |
pricebook_name | contact.pricebook_name |
default_templates | contact.default_templates |
associated_with_square | contact.associated_with_square |
can_add_card | contact.can_add_card |
can_add_bank_account | contact.can_add_bank_account |
cards | contact.cards |
checks | contact.checks |
bank_accounts | contact.bank_accounts |
vpa_list | contact.vpa_list |
notes | contact.notes |
created_time | contact.created_time |
last_modified_time | contact.last_modified_time |
tags | contact.tags |
zohopeople_client_id | contact.zohopeople_client_id |
Credit Note
Credit Note details
This method lets you fetch the details of an creditnote. This can be used only when the widget is displayed in the creditnotes details or creation page.
To fetch the details of an creditnote:
ZFAPPS.get('creditnote').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"creditnote": {
"creditnote_id": "112233",
"creditnote_number": "INV-000006",
"salesorder_id": "",
"salesorder_number": "",
"crm_owner_id": "",
"zcrm_potential_id": "",
"zcrm_potential_name": "",
"date": "2018-08-25",
"date_formatted": "25 Aug 2019",
"status": "paid",
"status_formatted": "Paid",
"amount": 101,
"amount_formatted": "$101.00",
.......
}
}
Credit Note list
This method lets you fetch the list of creditnotes. This can be used only when the widget is displayed in the creditnotes list page.
ZFAPPS.get('creditnotes').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"creditnotes": [
{
"creditnote_id": "112233",
"creditnote_number": "INV-000006",
...
},
{
"creditnote_id": "112233",
"creditnote_number": "INV-000006",
...
}
....
]
}
Supported Keys:
Property | Request |
---|---|
adjustment | creditnote.adjustment |
adjustment_description | creditnote.adjustment_description |
adjustment_formatted | creditnote.adjustment_formatted |
approver_id | creditnote.approver_id |
approvers_list | creditnote.approvers_list |
balance | creditnote.balance |
balance_formatted | creditnote.balance_formatted |
billing_address | creditnote.billing_address |
color_code | creditnote.color_code |
comments | creditnote.comments |
contact_category | creditnote.contact_category |
contact_persons | creditnote.contact_persons |
created_by_id | creditnote.created_by_id |
created_time | creditnote.created_time |
creditnote_id | creditnote.creditnote_id |
creditnote_number | creditnote.creditnote_number |
creditnote_refunds | creditnote.creditnote_refunds |
currency_code | creditnote.currency_code |
currency_id | creditnote.currency_id |
currency_symbol | creditnote.currency_symbol |
current_sub_status | creditnote.current_sub_status |
current_sub_status_formatted | creditnote.current_sub_status_formatted |
current_sub_status_id | creditnote.current_sub_status_id |
custom_field_hash | creditnote.custom_field_hash |
custom_fields | creditnote.custom_fields |
customer_id | creditnote.customer_id |
customer_name | creditnote.customer_name |
date | creditnote.date |
date_formatted | creditnote.date_formatted |
discount | creditnote.discount |
discount_applied_on_amount | creditnote.discount_applied_on_amount |
discount_type | creditnote.discount_type |
documents | creditnote.documents |
ewaybills | creditnote.ewaybills |
exchange_rate | creditnote.exchange_rate |
html_string | creditnote.html_string |
invoice_id | creditnote.invoice_id |
invoices_credited | creditnote.invoices_credited |
is_discount_before_tax | creditnote.is_discount_before_tax |
is_emailed | creditnote.is_emailed |
is_eway_bill_required | creditnote.is_eway_bill_required |
is_inclusive_tax | creditnote.is_inclusive_tax |
is_pre_gst | creditnote.is_pre_gst |
is_square_transaction | creditnote.is_square_transaction |
last_modified_by_id | creditnote.last_modified_by_id |
last_modified_time | creditnote.last_modified_time |
line_items | creditnote.line_items |
notes | creditnote.notes |
orientation | creditnote.orientation |
page_height | creditnote.page_height |
page_width | creditnote.page_width |
price_precision | creditnote.price_precision |
reference_number | creditnote.reference_number |
roundoff_value | creditnote.roundoff_value |
roundoff_value_formatted | creditnote.roundoff_value_formatted |
salesperson_id | creditnote.salesperson_id |
salesperson_name | creditnote.salesperson_name |
shipping_address | creditnote.shipping_address |
shipping_charge | creditnote.shipping_charge |
shipping_charge_exclusive_of_tax | creditnote.shipping_charge_exclusive_of_tax |
shipping_charge_exclusive_of_tax_formatted | creditnote.shipping_charge_exclusive_of_tax_formatted |
shipping_charge_formatted | creditnote.shipping_charge_formatted |
shipping_charge_inclusive_of_tax | creditnote.shipping_charge_inclusive_of_tax |
shipping_charge_inclusive_of_tax_formatted | creditnote.shipping_charge_inclusive_of_tax_formatted |
shipping_charge_sac_code | creditnote.shipping_charge_sac_code |
shipping_charge_tax | creditnote.shipping_charge_tax |
shipping_charge_tax_exemption_code | creditnote.shipping_charge_tax_exemption_code |
shipping_charge_tax_exemption_id | creditnote.shipping_charge_tax_exemption_id |
shipping_charge_tax_formatted | creditnote.shipping_charge_tax_formatted |
shipping_charge_tax_id | creditnote.shipping_charge_tax_id |
shipping_charge_tax_name | creditnote.shipping_charge_tax_name |
shipping_charge_tax_percentage | creditnote.shipping_charge_tax_percentage |
shipping_charge_tax_type | creditnote.shipping_charge_tax_type |
status | creditnote.status |
status_formatted | creditnote.status_formatted |
sub_statuses | creditnote.sub_statuses |
sub_total | creditnote.sub_total |
sub_total_formatted | creditnote.sub_total_formatted |
sub_total_inclusive_of_tax | creditnote.sub_total_inclusive_of_tax |
sub_total_inclusive_of_tax_formatted | creditnote.sub_total_inclusive_of_tax_formatted |
subject_content | creditnote.subject_content |
submitted_by | creditnote.submitted_by |
submitted_date | creditnote.submitted_date |
submitted_date_formatted | creditnote.submitted_date_formatted |
submitter_id | creditnote.submitter_id |
tax_rounding | creditnote.tax_rounding |
tax_total | creditnote.tax_total |
tax_total_formatted | creditnote.tax_total_formatted |
taxes | creditnote.taxes |
template_id | creditnote.template_id |
template_name | creditnote.template_name |
template_type | creditnote.template_type |
template_type_formatted | creditnote.template_type_formatted |
terms | creditnote.terms |
total | creditnote.total |
total_credits_used | creditnote.total_credits_used |
total_formatted | creditnote.total_formatted |
total_refunded_amount | creditnote.total_refunded_amount |
transaction_rounding_type | creditnote.transaction_rounding_type |
Salesorder
Salesorder details
This method lets you fetch the details of an salesorder. This can be used only when the widget is displayed in the salesorders details or creation page.
To fetch the details of an salesorder:
ZFAPPS.get('salesorder').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"salesorder": {
"salesorder_id": "112233",
"salesorder_number": "INV-000006",
"salesorder_id": "",
"salesorder_number": "",
"crm_owner_id": "",
"zcrm_potential_id": "",
"zcrm_potential_name": "",
"date": "2018-08-25",
"date_formatted": "25 Aug 2019",
"status": "paid",
"status_formatted": "Paid",
"amount": 101,
"amount_formatted": "$101.00",
.......
}
}
This method lets you fetch the list of salesorders. This can be used only when the widget is displayed in the salesorders list page.
ZFAPPS.get('retainerinvoice').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"salesorders": [
{
"salesorder_id": "112233",
"salesorder_number": "INV-000006",
...
},
{
"salesorder_id": "112233",
"salesorder_number": "INV-000006",
...
}
....
]
}
Supported Keys:
Property | Request |
---|---|
adjustment | salesorder.adjustment |
adjustment_description | salesorder.adjustment_description |
adjustment_formatted | salesorder.adjustment_formatted |
approver_id | salesorder.approver_id |
approvers_list | salesorder.approvers_list |
attachment_name | salesorder.attachment_name |
balance | salesorder.balance |
balance_formatted | salesorder.balance_formatted |
bcy_adjustment | salesorder.bcy_adjustment |
bcy_discount_total | salesorder.bcy_discount_total |
bcy_shipping_charge | salesorder.bcy_shipping_charge |
bcy_sub_total | salesorder.bcy_sub_total |
bcy_tax_total | salesorder.bcy_tax_total |
bcy_total | salesorder.bcy_total |
billing_address | salesorder.billing_address |
billing_address_id | salesorder.billing_address_id |
comments | salesorder.comments |
contact_category | salesorder.contact_category |
contact_persons | salesorder.contact_persons |
created_by_email | salesorder.created_by_email |
created_by_id | salesorder.created_by_id |
created_date | salesorder.created_date |
created_date_formatted | salesorder.created_date_formatted |
created_time | salesorder.created_time |
created_time_formatted | salesorder.created_time_formatted |
crm_custom_reference_id | salesorder.crm_custom_reference_id |
crm_owner_id | salesorder.crm_owner_id |
currency_code | salesorder.currency_code |
currency_id | salesorder.currency_id |
currency_symbol | salesorder.currency_symbol |
custom_fields | salesorder.custom_fields |
customer_id | salesorder.customer_id |
customer_name | salesorder.customer_name |
date | salesorder.date |
date_formatted | salesorder.date_formatted |
delivery_method | salesorder.delivery_method |
delivery_method_id | salesorder.delivery_method_id |
discount | salesorder.discount |
discount_applied_on_amount | salesorder.discount_applied_on_amount |
discount_percent | salesorder.discount_percent |
discount_total | salesorder.discount_total |
discount_total_formatted | salesorder.discount_total_formatted |
discount_type | salesorder.discount_type |
documents | salesorder.documents |
estimate_id | salesorder.estimate_id |
exchange_rate | salesorder.exchange_rate |
invoiced_status | salesorder.invoiced_status |
invoiced_status_formatted | salesorder.invoiced_status_formatted |
invoices | salesorder.invoices |
is_adv_tracking_in_package | salesorder.is_adv_tracking_in_package |
is_discount_before_tax | salesorder.is_discount_before_tax |
is_emailed | salesorder.is_emailed |
is_fba_shipment_created | salesorder.is_fba_shipment_created |
is_inclusive_tax | salesorder.is_inclusive_tax |
is_offline_payment | salesorder.is_offline_payment |
is_pre_gst | salesorder.is_pre_gst |
last_modified_by_id | salesorder.last_modified_by_id |
last_modified_time | salesorder.last_modified_time |
last_modified_time_formatted | salesorder.last_modified_time_formatted |
line_items | salesorder.line_items |
merchant_id | salesorder.merchant_id |
merchant_name | salesorder.merchant_name |
notes | salesorder.notes |
order_status | salesorder.order_status |
order_status_formatted | salesorder.order_status_formatted |
paid_status | salesorder.paid_status |
paid_status_formatted | salesorder.paid_status_formatted |
payment_terms | salesorder.payment_terms |
payment_terms_label | salesorder.payment_terms_label |
price_precision | salesorder.price_precision |
purchaseorders | salesorder.purchaseorders |
reference_number | salesorder.reference_number |
refundable_amount | salesorder.refundable_amount |
roundoff_value | salesorder.roundoff_value |
roundoff_value_formatted | salesorder.roundoff_value_formatted |
salesorder_id | salesorder.salesorder_id |
salesorder_number | salesorder.salesorder_number |
salesperson_id | salesorder.salesperson_id |
salesperson_name | salesorder.salesperson_name |
shipment_date | salesorder.shipment_date |
shipment_date_formatted | salesorder.shipment_date_formatted |
shipping_address | salesorder.shipping_address |
shipping_address_id | salesorder.shipping_address_id |
shipping_charge | salesorder.shipping_charge |
shipping_charge_exclusive_of_tax | salesorder.shipping_charge_exclusive_of_tax |
shipping_charge_exclusive_of_tax_formatted | salesorder.shipping_charge_exclusive_of_tax_formatted |
shipping_charge_formatted | salesorder.shipping_charge_formatted |
shipping_charge_inclusive_of_tax | salesorder.shipping_charge_inclusive_of_tax |
shipping_charge_inclusive_of_tax_formatted | salesorder.shipping_charge_inclusive_of_tax_formatted |
shipping_charge_sac_code | salesorder.shipping_charge_sac_code |
shipping_charge_tax | salesorder.shipping_charge_tax |
shipping_charge_tax_exemption_code | salesorder.shipping_charge_tax_exemption_code |
shipping_charge_tax_exemption_id | salesorder.shipping_charge_tax_exemption_id |
shipping_charge_tax_formatted | salesorder.shipping_charge_tax_formatted |
shipping_charge_tax_id | salesorder.shipping_charge_tax_id |
shipping_charge_tax_name | salesorder.shipping_charge_tax_name |
shipping_charge_tax_percentage | salesorder.shipping_charge_tax_percentage |
shipping_charge_tax_type | salesorder.shipping_charge_tax_type |
source | salesorder.source |
status | salesorder.status |
status_formatted | salesorder.status_formatted |
sub_total | salesorder.sub_total |
sub_total_exclusive_of_discount | salesorder.sub_total_exclusive_of_discount |
sub_total_exclusive_of_discount_formatted | salesorder.sub_total_exclusive_of_discount_formatted |
sub_total_formatted | salesorder.sub_total_formatted |
sub_total_inclusive_of_tax | salesorder.sub_total_inclusive_of_tax |
sub_total_inclusive_of_tax_formatted | salesorder.sub_total_inclusive_of_tax_formatted |
submitted_by | salesorder.submitted_by |
submitted_date | salesorder.submitted_date |
submitted_date_formatted | salesorder.submitted_date_formatted |
submitter_id | salesorder.submitter_id |
tax_rounding | salesorder.tax_rounding |
tax_total | salesorder.tax_total |
tax_total_formatted | salesorder.tax_total_formatted |
taxes | salesorder.taxes |
template_id | salesorder.template_id |
template_name | salesorder.template_name |
template_type | salesorder.template_type |
template_type_formatted | salesorder.template_type_formatted |
terms | salesorder.terms |
total | salesorder.total |
total_formatted | salesorder.total_formatted |
total_quantity | salesorder.total_quantity |
total_quantity_formatted | salesorder.total_quantity_formatted |
transaction_rounding_type | salesorder.transaction_rounding_type |
zcrm_potential_id | salesorder.zcrm_potential_id |
zcrm_potential_name | salesorder.zcrm_potential_name |
Retainer Invoice
Retainer Invoice details
This method lets you fetch the details of an retainerinvoice. This can be used only when the widget is displayed in the retainerinvoices details or creation page.
To fetch the details of an retainerinvoice:
ZFAPPS.get('retainerinvoice').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"retainerinvoice": {
"retainerinvoice_id": "112233",
"retainerinvoice_number": "INV-000006",
"salesorder_id": "",
"salesorder_number": "",
"crm_owner_id": "",
"zcrm_potential_id": "",
"zcrm_potential_name": "",
"date": "2018-08-25",
"date_formatted": "25 Aug 2019",
"status": "paid",
"status_formatted": "Paid",
"amount": 101,
"amount_formatted": "$101.00",
.......
}
}
This method lets you fetch the list of retainerinvoices. This can be used only when the widget is displayed in the retainerinvoices list page.
ZFAPPS.get('retainerinvoice').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"retainerinvoices": [
{
"retainerinvoice_id": "112233",
"retainerinvoice_number": "INV-000006",
...
},
{
"retainerinvoice_id": "112233",
"retainerinvoice_number": "INV-000006",
...
}
....
]
}
Supported Keys:
Property | Request |
---|---|
retainerinvoice_id | retainerinvoice.retainerinvoice_id |
retainerinvoice_number | retainerinvoice.retainerinvoice_number |
date | retainerinvoice.date |
date_formatted | retainerinvoice.date_formatted |
status | retainerinvoice.status |
status_formatted | retainerinvoice.status_formatted |
last_payment_date | retainerinvoice.last_payment_date |
last_payment_date_formatted | retainerinvoice.last_payment_date_formatted |
reference_number | retainerinvoice.reference_number |
customer_id | retainerinvoice.customer_id |
roundoff_value | retainerinvoice.roundoff_value |
roundoff_value_formatted | retainerinvoice.roundoff_value_formatted |
transaction_rounding_type | retainerinvoice.transaction_rounding_type |
vat_treatment | retainerinvoice.vat_treatment |
place_of_supply | retainerinvoice.place_of_supply |
place_of_supply_formatted | retainerinvoice.place_of_supply_formatted |
customer_name | retainerinvoice.customer_name |
contact_persons | retainerinvoice.contact_persons |
currency_id | retainerinvoice.currency_id |
currency_code | retainerinvoice.currency_code |
currency_symbol | retainerinvoice.currency_symbol |
exchange_rate | retainerinvoice.exchange_rate |
is_viewed_by_client | retainerinvoice.is_viewed_by_client |
unused_retainer_payments | retainerinvoice.unused_retainer_payments |
unused_retainer_payments_formatted | retainerinvoice.unused_retainer_payments_formatted |
client_viewed_time | retainerinvoice.client_viewed_time |
client_viewed_time_formatted | retainerinvoice.client_viewed_time_formatted |
is_inclusive_tax | retainerinvoice.is_inclusive_tax |
tax_rounding | retainerinvoice.tax_rounding |
line_items | retainerinvoice.line_items |
sub_total | retainerinvoice.sub_total |
sub_total_formatted | retainerinvoice.sub_total_formatted |
total | retainerinvoice.total |
total_formatted | retainerinvoice.total_formatted |
taxes | retainerinvoice.taxes |
payment_made | retainerinvoice.payment_made |
payment_made_formatted | retainerinvoice.payment_made_formatted |
payment_drawn | retainerinvoice.payment_drawn |
payment_drawn_formatted | retainerinvoice.payment_drawn_formatted |
balance | retainerinvoice.balance |
balance_formatted | retainerinvoice.balance_formatted |
allow_partial_payments | retainerinvoice.allow_partial_payments |
price_precision | retainerinvoice.price_precision |
payment_options | retainerinvoice.payment_options |
is_emailed | retainerinvoice.is_emailed |
contact_persons_associated | retainerinvoice.contact_persons_associated |
payments | retainerinvoice.payments |
payment_refunds | retainerinvoice.payment_refunds |
documents | retainerinvoice.documents |
comments | retainerinvoice.comments |
billing_address | retainerinvoice.billing_address |
shipping_address | retainerinvoice.shipping_address |
notes | retainerinvoice.notes |
terms | retainerinvoice.terms |
custom_fields | retainerinvoice.custom_fields |
template_id | retainerinvoice.template_id |
template_name | retainerinvoice.template_name |
template_type | retainerinvoice.template_type |
template_type_formatted | retainerinvoice.template_type_formatted |
created_time | retainerinvoice.created_time |
last_modified_time | retainerinvoice.last_modified_time |
created_by_id | retainerinvoice.created_by_id |
last_modified_by_id | retainerinvoice.last_modified_by_id |
attachment_name | retainerinvoice.attachment_name |
invoice_url | retainerinvoice.invoice_url |
submitted_date | retainerinvoice.submitted_date |
submitted_date_formatted | retainerinvoice.submitted_date_formatted |
submitted_by | retainerinvoice.submitted_by |
approvers_list | retainerinvoice.approvers_list |