Getting started with ZeptoMail
Zoho ZeptoMail is a reliable, secure and scalable transactional email service. We do one thing and one thing well—transactional email sending. With an exclusive focus on transactional emails, we take care of your important emails while you focus on your business.
Choose how you want to send emails from ZeptoMail
- SMTP Relay
- Email API
SMTP Relay: Fast and easy setup
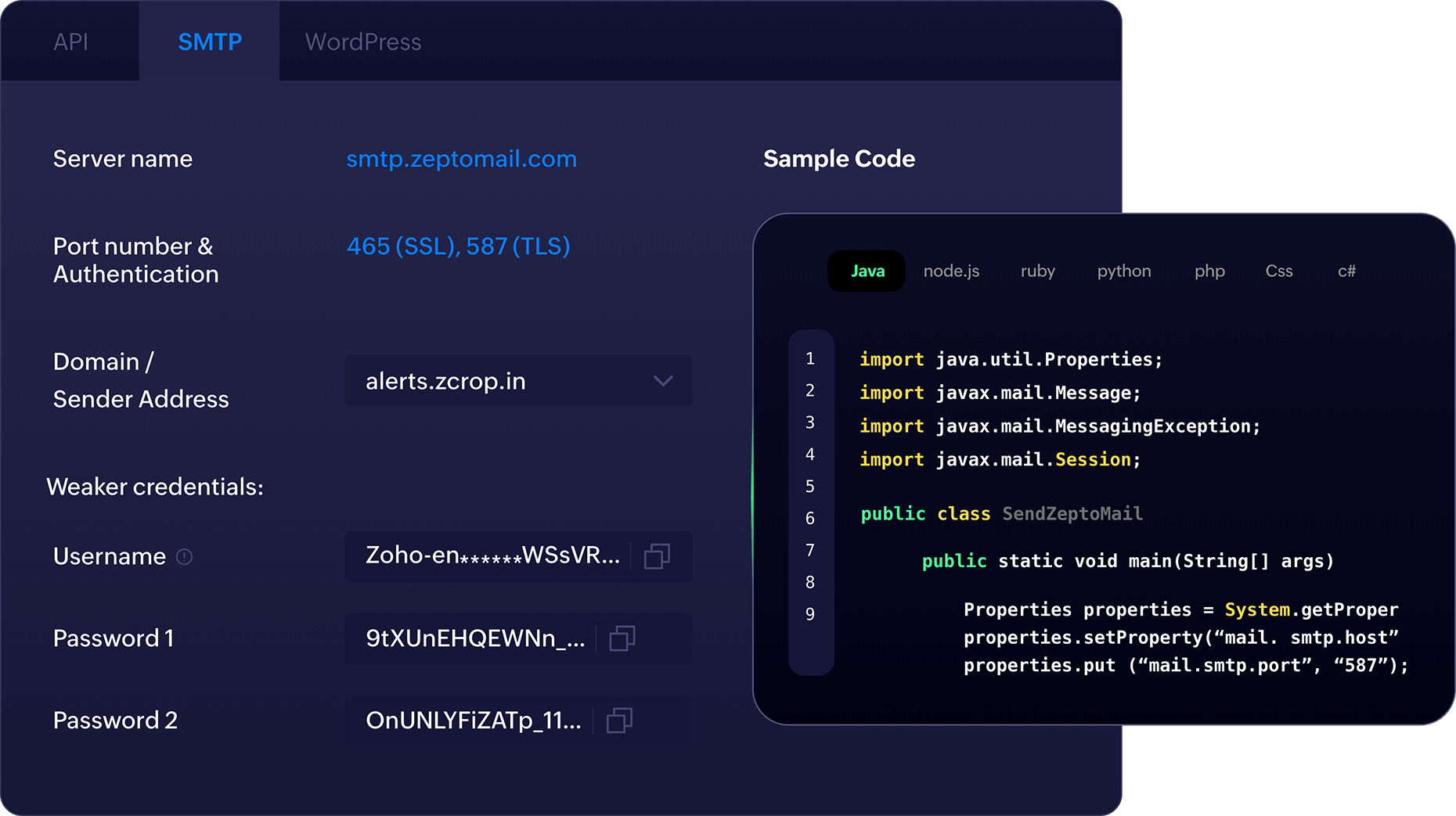

What is SMTP?
Simple Mail Transfer Protocol (SMTP) is the standard protocol used to send, receive, or relay outgoing messages between email senders and receivers. It is the most commonly used protocol for email sending.
When should you use SMTP?
If you're already using SMTP in your application or only need a simple integration, ZeptoMail's SMTP relay is the quickest way to get you started. With SMTP, you can do away with the bells and whistles to integrate your application in just a few steps.
Sending emails using SMTP
Using SMTP relay to send your transactional emails is as simple as copying credentials from ZeptoMail and entering it into your application or platform.
Track Recipient Activity
Use metadata to track opens and clicks on emails sent using SMTP. You can then view this data in your ZeptoMail account or use webhooks to get instant notifications.
Secure Connection
Security is one of the cornerstones of ZeptoMail. To ensure complete safety of your email data, we only support TLS v1.2 for data transfer between the application and servers.
Email APIs: Deeper integration
curl "https://zeptomail.zoho.com/v1.1/email" \
-X POST \
-H "Accept: application/json" \
-H "Content-Type: application/json" \
-H "Authorization: [Authorization key]" \
-d '{
"from": {"address": "yourname@yourdomain.com"},
"to": [{
"email_address": {
"address": "receiver@yourdomain.com",
"name": "Receiver"
}
}],
"subject":"Test Email",
"htmlbody":" Test email sent successfully. "}'
// https://www.npmjs.com/package/zeptomail
// For ES6
import { SendMailClient } from "zeptomail";
// For CommonJS
// var { SendMailClient } = require("zeptomail");
const url = "zeptomail.zoho.com/";
const token = "[Authorization key]";
let client = new SendMailClient({ url, token });
client
.sendMail({
from: {
address: "yourname@yourdomain.com",
name: "noreply"
},
to: [
{
email_address: {
address: "receiver@yourdomain.com",
name: "Receiver"
},
},
],
subject: "Test Email",
htmlbody: " Test email sent successfully.",
})
.then((resp) => console.log("success"))
.catch((error) => console.log("error"));
using System;
using System.Net;
using System.Text;
using System.IO;
using System.Net.Http;
using Newtonsoft.Json;
using Newtonsoft.Json.Linq;
namespace Rextester {
public class Program {
public static void Main(string[] args) {
System.Net.ServicePointManager.SecurityProtocol =
System.Net.SecurityProtocolType.Tls12;
var baseAddress = "https://zeptomail.zoho.com/v1.1/email";
var http = (HttpWebRequest)WebRequest.Create(new Uri(baseAddress));
http.Accept = "application/json";
http.ContentType = "application/json";
http.Method = "POST";
http.PreAuthenticate = true;
http.Headers.Add("Authorization", "[Authorization key]");
JObject parsedContent = JObject.Parse("{"+
"'from': {'address': 'yourname@yourdomain.com'},"+
"'to': [{'email_address': {"+
"'address': 'receiver@yourdomain.com',"+
"'name': 'Receiver'"+
"}}],"+
"'subject':'Test Email',"+
"'htmlbody':' Test email sent successfully.'"+
"}");
Console.WriteLine(parsedContent.ToString());
ASCIIEncoding encoding = new ASCIIEncoding();
Byte[] bytes = encoding.GetBytes(parsedContent.ToString());
Stream newStream = http.GetRequestStream();
newStream.Write(bytes, 0, bytes.Length);
newStream.Close();
var response = http.GetResponse();
var stream = response.GetResponseStream();
var sr = new StreamReader(stream);
var content = sr.ReadToEnd();
Console.WriteLine(content);
}
}
}
import requests
url = "https://zeptomail.zoho.com/v1.1/email"
payload = """{
"from": {
"address": "yourname@yourdomain.com"
},
"to": [{
"email_address": {
"address": "receiver@yourdomain.com",
"name": "Receiver"
}}],
"subject":"Test Email",
"htmlbody":"Test email sent successfully."
}"""
headers = {
'accept': "application/json",
'content-type': "application/json",
'authorization': "[Authorization key]",
}
response = requests.request("POST",url,data=payload,headers=headers)
print(response.text)
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://zeptomail.zoho.com/v1.1/email",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_SSLVERSION => CURL_SSLVERSION_TLSv1_2,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => '{
"from": { "address": "yourname@yourdomain.com"},
"to": [
{
"email_address": {
"address": "receiver@yourdomain.com",
"name": "Receiver"
}
}
],
"subject":"Test Email",
"htmlbody":" Test email sent successfully. ",
}',
CURLOPT_HTTPHEADER => array(
"accept: application/json",
"authorization: [Authorization key]",
"cache-control: no-cache",
"content-type: application/json",
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
?>
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import org.json.JSONObject;
public class JavaSendapi {
public static void main(String[] args) throws Exception {
String postUrl = "https://zeptomail.zoho.com/v1.1/email";
BufferedReader br = null;
HttpURLConnection conn = null;
String output = null;
StringBuffer sb = new StringBuffer();
try {
URL url = new URL(postUrl);
conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
conn.setRequestProperty("Accept", "application/json");
conn.setRequestProperty("Authorization", "[Authorization key]");
JSONObject object = new JSONObject("{\n" +
" \"from\": {\n" +
" \"address\": \"yourname@yourdomain.com\"\n" +
" },\n" +
" \"to\": [\n" +
" {\n" +
" \"email_address\": {\n" +
" \"address\": \"receiver@yourdomain.com\",\n" +
" \"name\": \"Receiver\"\n" +
" }\n" +
" }\n" +
" ],\n" +
" \"subject\": \"Test Email\",\n" +
" \"htmlbody\": \" Test email sent successfully.\"\n" +
"}");
OutputStream os = conn.getOutputStream();
os.write(object.toString().getBytes());
os.flush();
br = new BufferedReader(
new InputStreamReader((conn.getInputStream()))
);
while ((output = br.readLine()) != null) {
sb.append(output);
}
System.out.println(sb.toString());
} catch (Exception e) {
br = new BufferedReader(
new InputStreamReader((conn.getErrorStream()))
);
while ((output = br.readLine()) != null) {
sb.append(output);
}
System.out.println(sb.toString());
} finally {
try {
if (br != null) {
br.close();
}
} catch (Exception e) {
e.printStackTrace();
}
try {
if (conn != null) {
conn.disconnect();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
What are APIs?
APIs enable a connection between your application and ZeptoMail. It gives applications access to the functionality available in ZeptoMail, such as transactional emails sending, using templates, and uploading files.Our email APIs come with client libraries for multiple languages like Curl, C#, Python, Node JS and more.
When should you use email API?
If your application requires deeper insight into the performance of your emails, APIs are exactly what you need. Use ZeptoMail's APIs to easily integrate, view, and retrieve information.
Sending emails using email API
If you're familiar with programming languages and require deeper integration with ZeptoMail, you can use our email APIs to send emails from the platform. APIs go beyond just email sending.
Email templates at the ready
Pick from the multiple sample templates already available in ZeptoMail. Customize these templates or even build your own from scratch. Use the APIs to send single or batch emails using templates.
Robust API libraries
ZeptoMail provides email API is most common programming languages to make the integration easy and hassle-free.
Separate your email streams
Managing multiple applications or sending a variety of transactional emails from your business? Group your emails by type, purpose, app, and more using Mail Agents. Each Mail Agent has a unique API token and SMTP credentials
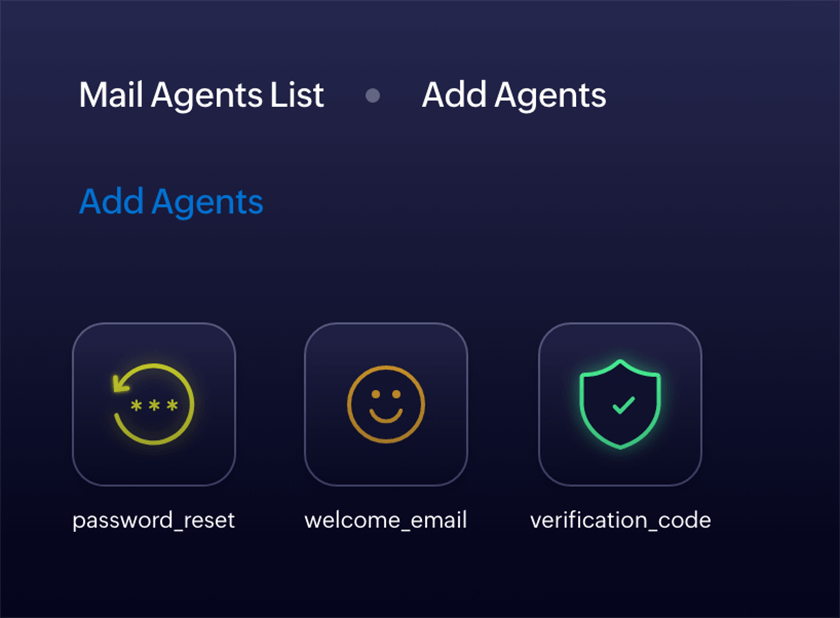
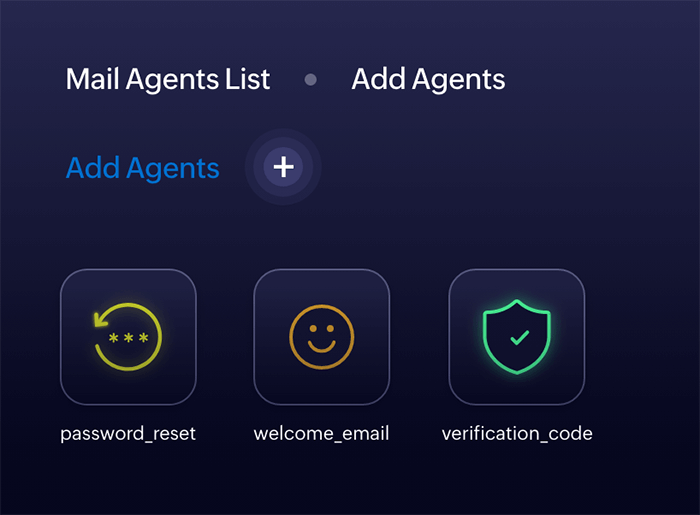
Great deliverability
No matter how you choose to integrate your application with ZeptoMail, great email deliverability is guaranteed. ZeptoMail's processes are optimized to ensure good inbox placement and fast delivery.
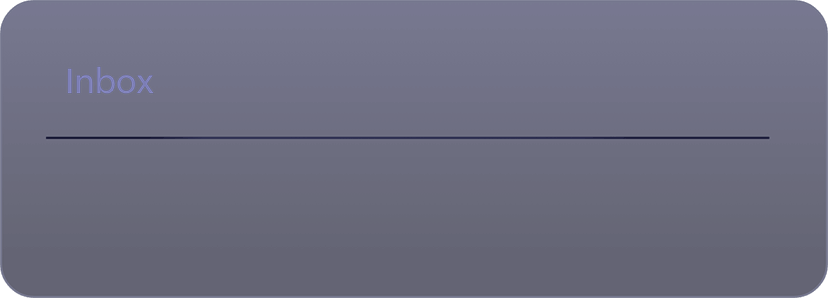
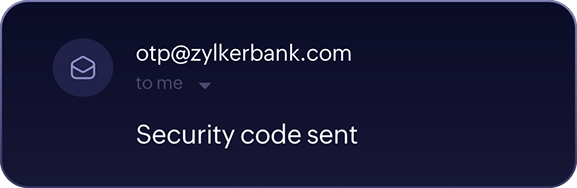
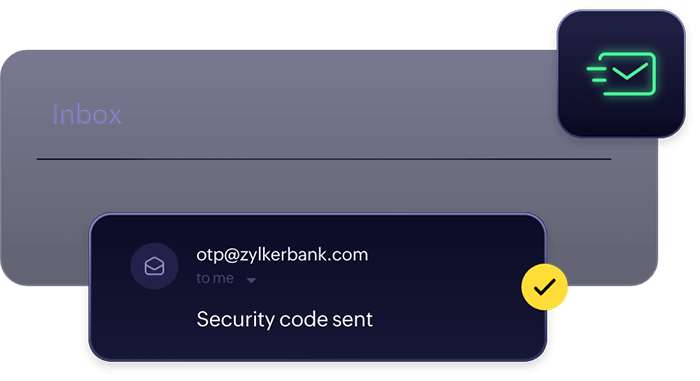
Developer-friendly documentation
Comprehensive and developer-friendly help documentation helps you make the most of our service. Simply follow the instructions to get started with ZeptoMail and navigate the platform.
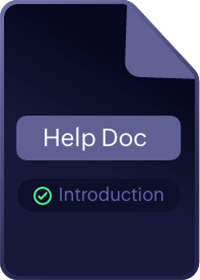
You’re all set to start sending emails!
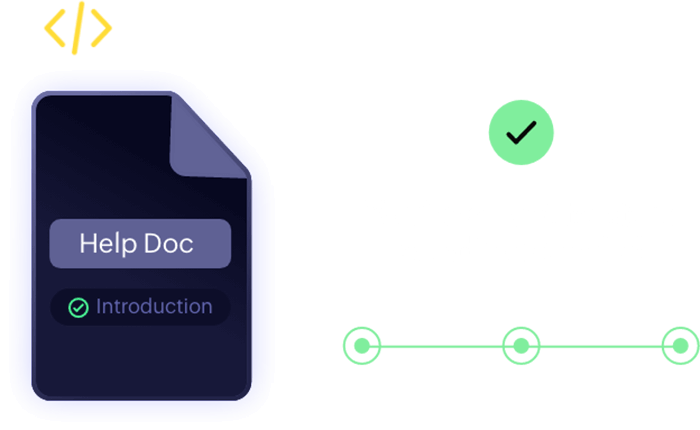
Extensive support
Uninterrupted email sending with good deliverability is our priority. We have a knowledgable and capable technical assistance team available around the clock to provide extensive support.
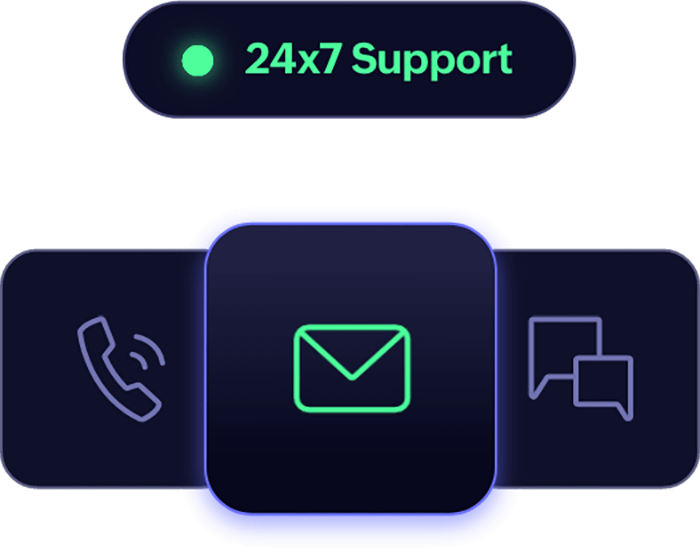